|
CLASS-XII
COMPUTER SCIENCE
(Subject Code 083)
SAMPLE PAPER 2014 - 15 |
|
Time
allowed : 3 hours |
|
Maximum
Marks: 70 |
|
Instructions:
(i) All questions are compulsory.
(ii) Programming Language: Section A C+ +.
(iii) Programming Language : Section B Python.
(iv) Answer either Section A or B, and Section C is compulsory. |
|
Section
A (C++) |
|
|
Q1 (a) |
Differentiate between ordinary
function and member functions in C++. Explain with an example. |
[2] |
(b) |
Write the related library
function name based upon the given information in C++.
(i) Get single character using keyboard. This function is available in
stdio.h file.
(ii) To check whether given character is alpha numeric character or
not. This function is available in ctype.h file. |
[1] |
(c) |
Rewrite the following C++
program after removing all the syntactical errors (if any), underlining
each correction. :
include <iostream.h>
#define PI=3.14
void main( )
{ float r;a;
cout<<’enter any radius’;
cin>>r;
a=PI*pow(r,2);
cout<< ”Area=” << a
}
|
[2] |
(d) |
Write the output from the
following C++ program code:
#include <iostream.h>
#include <ctype.h>
void strcon(char s[])
{
for(int i=0,l=0;s[i]!='\0';i++,l++);
for(int j=0; j<l; j++)
{
if (isupper(s[j]))
s[j]=tolower(s[j])+2;
else if ( islower(s[j]))
s[j]=toupper(s[j])-2;
else
s[j]='@';
}
}
void main()
{
char *c="Romeo Joliet";
strcon(c);
cout<<"Text= "<<c<<endl;
c=c+3;
cout<<"New Text= "<<c<<endl;
c=c+5-2;
cout<<"last Text= "<<c
}
|
[2]
|
(e) |
Find the output of the following
C++ program:
#include<iostream.h>
#include<conio.h>
#include<ctype.h>
class Class
{
int Cno,total;
char section;
public:
Class(int no=1)
{
Cno=no;
section='A';
total=30;
}
void addmission(int c=20)
{
section++;
total+=c;
}
void ClassShow()
{
cout<<Cno<<":"<<section<<":"<<total<<endl;
}
};
void main()
{
Class C1(5),C2;
C1.addmission(25);
C1.ClassShow();
C2.addmission();
C1.addmission(30);
C2.ClassShow();
C1.ClassShow();
}
|
|
(f) |
Study the following C++ program
and select the possible output(s) from it :
Find the maximum and minimum value of L.
#include<stdlib.h>
#include<iostream.h>
#include<string.h>
void main()
{
randomize();
char P[]="C++PROGRAM";
long L;
for(int I=0;P[I]!='R';I++)
{
L=random (sizeof(L)) +5;
cout<<P[L]<<"-";
}
}
i) R-P-O-R-
ii) P-O-R-+-
iii)O-R-A-G-
iv) A-G-R-M-
|
|
Q2(a) |
How encapsulation and
abstraction are implemented in C++ language? Explain with an example. |
[2] |
(b) |
Answer the questions (i) and
(ii) after going through the following C++ class:
class Stream
{
int StreamCode ; char Streamname[20];float fees;
public:
Stream( ) //Function 1
{StreamCode=1; strcpy (Streamname,"DELHI"); fees=1000;}
void display(float C) //Function 2
{
cout<<StreamCode<<":"<<Streamname<<":"<<fees<<endl;
}
~Stream( ) //Function 3
{
cout<<"End of Stream Object"<<endl;
}
Stream (int SC,char S[ ],float F) ; //Function 4
};
i) In Object Oriented
Programming, what are Function 1 and Function 4 combined together
referred as? Write the definition of function 4.
ii) What is the
difference between the following statements?
Stream S(11,”Science”,8700);
Stream S=Stream(11,”Science”,8700); |
|
(c) |
Define a class Customer with the
following specifications.
Private Members :
Customer_no integer
Customer_name char (20)
Qty integer
Price, TotalPrice, Discount, Netprice float
Member Functions:
Public members:
* A constructer to assign initial values of
Customer_no as 111,Customer_name as “Leena”, Quantity as 0 and Price,
Discount and Netprice as 0.
* Input( ) – to read data members(Customer_no,
Customer_name, Quantity and Price) call Caldiscount().
* Caldiscount ( ) – To calculate Discount according
to TotalPrice and NetPrice
TotalPrice = Price*Qty
TotalPrice >=50000 – Discount
25% of TotalPrice
TotalPrice >=25000 and
TotalPrice <50000 - Discount 15% of TotalPrice
TotalPrice <250000 - Discount
10% of TotalPrice
Netprice= TotalPrice-Discount
*Show( ) – to display Customer details. |
[4] |
(d) |
Answer the questions (i) to (iv)
based on the following code:
class AC
{
char Model[10];
char Date_of_purchase[10];
char Company[20];
public( );
AC( );
void entercardetail( );
void showcardetail( );
};
class Accessories : protected AC
{
protected:
char Stabilizer[30];
char AC_cover[20];
public:
float Price;
Accessories( );
void enteraccessoriesdetails( );
void showaccessoriesdetails( );
};
class Dealer : public Accessories
{
int No_of_dealers;
char dealers_name[20];
int No_of_products;
public:
Dealer( );
void enterdetails( );
void showdetails( );
};
(i) How many bytes will be required by an object of class Dealer and
class Accessories?
(ii) Which type of inheritance is illustrated in the above c++ code?
Write the base class and derived class name of class Accessories.
(ii) Write names of all the members which are accessible from the
objects of class Dealer.
(iv) Write names of all the members accessible from member functions of
class Dealer. |
[4] |
Q3(a) |
An array T[-1..35][-2..15] is
stored in the memory along the row with each element occupying 4 bytes.
Find out the base address and address of element T[20][5], if an
element T[2][2] is stored at the memory location 3000. Find the total
number of elements stored in T and number of bytes allocated to T |
[3] |
(b) |
Write a function SORTSCORE() in
C++ to sort an array of structure IPL in descending order of score
using selection sort .
Note : Assume the following definition of structure IPL.
struct IPL
{
int Score;
char Teamname[20];
};
|
[3] |
(c) |
Write member functions to
perform POP and PUSH operations in a dynamically allocated stack
containing the objects of the following structure:
struct Game
{
char Gamename[30];
int numofplayer;
Game *next;
};
|
[4] |
(d) |
Write a function in C++ to print
the sum of all the non-negative elements present on both the diagonal
of a two dimensional array passed as the argument to the function. |
[2] |
(e) |
Evaluate the following postfix
expression. Show the status of stack after execution of each operation
separately:
2,13, + , 5, -,6,3,/,5,*,< |
[2] |
Q4(a) |
Write the command to place the
file pointer at the 10th and 4th record starting position using seekp()
or seekg() command. File object is ‘file’ and record name is ‘STUDENT’. |
[1] |
(b) |
Write a function in C++ to count
and display the no of three letter words in the file “VOWEL.TXT”.
Example:
If the file contains:
A boy is playing there. I love to eat pizza. A plane is in the sky.
Then the output should be: 4 |
[2] |
(c) |
Given the binary file CAR.Dat,
containing records of the following class CAR type:
class CAR
{
int C_No;
char C_Name[20];
float Milage;
public:
void enter( )
{
cin>> C_No ; gets(C_Name) ; cin >> Milage;
}
void display( )
{
cout<< C_No ; cout<<C_Name ; cout<< Milage;
}
int RETURN_Milage( )
{
return Milage;
}
};
Write a function in C++, that would read contents from the file CAR.DAT
and display the details of car with mileage between 100 to 150. |
[3] |
Section
B (Python) |
|
|
Q1(a) |
How is a static method different
from an instance method? |
[2] |
(b) |
Name the function / method
required for
i) Finding second occurrence of m in madam.
ii) get the position of an item in the list |
[1] |
(c) |
Rewrite the following python
code after removing all syntax error(s). Underline the corrections done.
def main():
r = raw-input(‘enter any radius : ‘)
a = pi * math.pow(r,2)
print “ Area = “ + a |
[2] |
(d) |
Give the output of following
with justification
x = 3
x+= x-x
print x |
[2] |
(e) |
What will be printed, when
following python code is executed
class person:
def __init__(self,id):
self.id = id
arjun = person(150)
arjun.__dict__[‘age’] = 50
print arjun.age + len(arjun.__dict__)
Justify your answer. |
[3] |
(f) |
What are the possible outcome(s)
expected from the following
python code? Also specify maximum and minimum value, which we can have.
def main():
p = 'MY PROGRAM'
i = 0
while p[i] != 'R':
l =
random.randint(0,3) + 5
print p[l],'-',
i += 1
i) R - P - O - R -
ii) P - O - R - Y -
iii) O -R - A - G -
iv) A- G - R - M - |
[2] |
Q2(a) |
How data encapsulation and data abstraction are implemented
in python, explain with example. |
[2] |
(b) |
What will following python code produce, justify your answer
[2]
x = 5
y = 0
print ‘A’
try :
print ‘B’
a = x / y
print ‘C’
except ZerorDivisionError:
print ‘F’
except :
print ‘D’
|
|
(c) |
Write a class customer in python having following
specifications
Instance attributes:
customernumber - numeric value
customername - string value
price, qty, discount, totalprice, netprice - numeric
value
methods :
init() to
assign initial values of customernumber as 111, customername as
“Leena”, qty as 0 and price, discount & netprice as 0.
caldiscount ( )
– To calculate discount, totalprice and netprice
totalprice = price * qty
discount is 25% of totalprice, if totalprice >=50000
discount 15% of totalprice, if totalprice >=25000 and totalprice
<50000
discount 10% of totalprice, if totalprice <250000
netprice= totalprice - discount
input( ) – to
read data members customername, customernumbar, price, qty and call
caldiscount() to calculate discount, totalprice and netprice.
show( ) – to
display Customer details. |
[4] |
(d) |
What are the different ways of overriding function call in
derived class of python ? Illustrate with example. |
[2] |
(e) |
Write a python function to find sum of square-root of
elements of a list. List is received as argument, and function returns
the sum. Ensure that your function is able to handle various situations
viz. list containing numbers & strings, module required is imported
etc. |
[2] |
Q3(a) |
What will be the status of following list after third pass of
bubble sort and third pass of selection sort used for arranging
elements in ascending order?
40, 67, -23, 11, 27, 38, -1 |
[3] |
(b) |
Write a python function to search for a value in the given
list using binary search method. Function should receive the list and
value to be searched as argument and return 1 if the value is found 0
otherwise. |
[2] |
(c) |
Define stack class in python to operate on stack of numbers. |
[4] |
(d) |
Write a python function using yield statement to generate
prime numbers till the value provided as parameter to it. |
[3] |
(e) |
Evaluate the following postfix expression. Show the status of
stack after execution of each operation separately:
2,13, + , 5, -,6,3,/,5,*,< |
[2] |
Q4(a) |
How is method write() different from writelines() in python? |
[1] |
(b) |
Given a pickled file - log.dat,
containing list of strings. Write a python function that reads the file
and looks for a line of the form
Xerror: 0.2395
whenever such line is encountered, extract the floating point value and
compute the total of these error values. When you reach end of file
print total number of such error lines and average of error value. |
[3] |
(c) |
Given a text file car.txt
containing following information of cars
carNo, carname, milage. Write a python function to display details of
all those cars whose milage is from 100 to 150. |
[2] |
Section C |
|
|
Q5 (a) |
Define degree and cardinality. Based upon given table write
degree and cardinality.
PATIENTS
PatNo |
PatName |
Dept |
DocID |
1 |
Leena |
ENT |
100 |
2 |
Surpreeth |
Ortho |
200 |
3 |
Madhu |
ENT |
100 |
4 |
Neha |
ENT |
100 |
5 |
Deepak |
Ortho |
200 |
|
[2] |
(b) |
Write SQL commands for the queries (i) to (iv) and output for
(v) & (viii) based on a table COMPANY and CUSTOMER
COMPANY
CID |
NAME |
CITY |
PRODUCTNAME |
111 |
SONY |
DELHI |
TV |
222 |
NOKIA |
MUMBAI |
MOBILE |
333 |
ONIDA |
DELHI |
TV |
444 |
SONY |
MUMBAI |
MOBILE |
555 |
BLACKBERRY |
MADRAS |
MOBILE |
666 |
DELL |
DELHI |
LAPTOP |
CUSTOMER
CUSTID |
NAME |
PRICE |
QTY |
CID |
101 |
Rohan Sharma |
70000 |
20 |
222 |
102 |
Deepak Kumar |
50000 |
10 |
666 |
103 |
Mohan Kumar |
30000 |
5 |
111 |
104 |
Sahil Bansak |
35000 |
3 |
333 |
105 |
Neha Soni |
25000 |
7 |
444 |
106 |
Sonal Aggarwal |
20000 |
5 |
333 |
107 |
Arjun Singh |
50000 |
15 |
666 |
(i) To display those company name which are having prize less than
30000.
(ii) To display the name of the companies in reverse alphabetical order.
(iii) To increase the prize by 1000 for those customer whose name
starts with ‘S’ (iv) To add one more column totalprice with
decimal(10,2) to the table customer
(v) SELECT COUNT(*) ,CITY FROM COMPANY GROUP BY CITY;
(vi) SELECT MIN(PRICE), MAX(PRICE) FROM CUSTOMER WHERE QTY>10 ;
(vii) SELECT AVG(QTY) FROM CUSTOMER WHERE NAME LIKE “%r%;
(viii) SELECT PRODUCTNAME,CITY, PRICE FROM COMPANY,CUSTOMER WHERE
COMPANY.CID=CUSTOMER.CID AND PRODUCTNAME=”MOBILE”; |
[6] |
Q6(a) |
State and define principle of Duality. Why is it so important
in Boolean Algebra? |
[2] |
(b) |
Write the equivalent boolean expression for the following
logic circuit
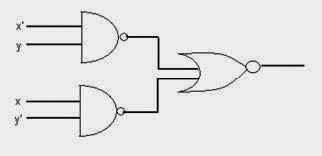
|
[2] |
(c) |
Write Product Of Sum expression of the function F (a,b,c,d)
from the given truth table
a |
b |
c |
d |
F |
0
0
0
0
0
0
0
0
1
1
1
1
1
1
1
1 |
0
0
0
0
1
1
1
1
0
0
0
0
1
1
1
1 |
0
0
1
1
0
0
1
1
0
0
1
1
0
0
1
1 |
0
1
0
1
0
1
0
1
0
1
0
1
0
1
0
1 |
0
0
1
1
0
1
1
0
0
0
1
1
0
0
0
1 |
|
[1] |
(d) |
Obtain the minimal SOP form for the following boolean
expression using K-Map.
F(w,x,y,z) = (0,2,3,5,7,8,10,11,13,15) |
[3] |
Q7(a) |
Give any two advantage of using Optical Fibres. |
[1] |
|
Indian School, in Mumbai is starting up the network between
its different wings. There are Four Buildings named as SENIOR, JUNIOR,
ADMIN and HOSTEL as shown below.:
SENIOR
JUNIOR
ADMIN
HOSTEL
The distance between various buildings is as follows:
ADMIN TO SENIOR 200m
ADMIN TO JUNIOR 150m
ADMIN TO HOSTEL 50m
SENIOR TO JUNIOR 250m
SENIOR TO HOSTEL 350m
JUNIOR TO HOSTEL 350m
Number of Computers in Each Building
SENIOR 130
JUNIOR 80
ADMIN 160
HOSTEL 50
(b1) Suggest the cable
layout of connections between the buildings.
(b2) Suggest the most
suitable place (i.e. building) to house the server of this School,
provide a suitable reason.
(b3) Suggest the
placement of the following devices with justification.
· Repeater
· Hub / Switch
(b4) The organization
also has Inquiry office in another city about 50-60 Km away in Hilly
Region. Suggest the suitable transmission media to interconnect to
school and Inquiry office out of the following .
· Fiber Optic Cable
· Microwave
· Radio Wave |
|
(c) |
Identify the Domain name and URL from the following.
http://www.income.in/home.aboutus.hml
|
[1] |
(d) |
What is Web Hosting? |
[1] |
(e) |
What is the difference between packet & message switching? |
[1] |
(f) |
Define firewall. |
[1] |
(g) |
Which protocol is used to creating a connection with a remote
machine? |
[1] |
|
|
|
No comments:
Post a Comment
We love to hear your thoughts about this post!
Note: only a member of this blog may post a comment.