Python - A Quick Revision
CBSE Class 12 - Computer Science / Informatics Practices
Q1: What is python?
Answer: Python is an object-oriented high-level computer language developed by Guido Van Rossum in 1990s. It was named after a British TV show namely ‘Monty Python’s Flying circus’.
Q2: What are the major advantages of Python language?
Answer: Python is a very powerful interpreted language (executes the code line by line at a time).
Some advantages of python are:
Easy to use
Expressive language
Cross-platform
Free and open sources
Q3: What does Python character set consist of?
Answer: Python character set consists:
Letters : A-Z,a-z
Digits : 0-9
Special symbols : space,+,-,*,/,** etc.
Whitespace : blank space, tabs(->),carriage return, newline, formfeed
Q4: What is a token?
Answer: The smallest individual unit in a program is known as a token. Python has the following tokens:
a) Keywords
b) Identifiers
c) Literals
d) Operators
e) Punctuators
Q5: What is a keyword in Python?
Answer: It is a word having special meaning and must not be used as a normal identifier. For example: if, while, int etc.
Q6: What is an identifier?
Answer: Identifiers are fundamental building blocks of a program and used to declare variable, objects, class, function etc.
Q7: What are the rules for defining an identifier?
Answer: Identifier’s forming rules are :
1) The first character must be a letter.
2) No special character except (_) is allowed.
3) Must not be a keyword.
4) Upper and lower case letters are different.
Q8: What are literals in Python language?
Answer: Literals are data items that have a fixed value. Different types of literals are:
1) Strings
2) Numeric
3) Boolean
4) Special literal- none
5) Literal collection
Q9: Write a short note on string literals in Python.
Answer: String literals are enclosed in quotes(either in single or double). Eg. ‘a’,’abc’,”x”,”xyz”
It also allows having certain nongraphic characters (those characters that cannot be typed directly from keyboard e.g. backspace, tabs, carriage return etc. )
There are two string types:
(A) single-line
(B) multi-line
Single line string is created by enclosing text in single quotes (‘ ’) or double quotes (“ ”) and must terminate in one line.
Multiline string: It can be created in two ways:
(a) By adding a backslash
eg: text1 = 'hello\
World'
(b) By adding a triple quotation mark
eg: str1 = '''hello
World.
There I come!!!
Cheers'''
Q10: What are the different types of numeric literals?
Answer: Numeric literal is of three types int, float and complex.
Q11: What are the different types of integer literals can be represented?
Answer: Integer literals are whole numbers without any fractional part. There are three types of integer literals.
Ⓐ Decimal integer literals:
A sequence of digit unless it begins with 0 is taken to be a decimal integer literal. Eg: 23 ,456 etc.
Ⓑ Octal integer literals:
A sequence of digit (only from 0-7, 8 & 9 are invalid) staring with 0 is an octal integer. Example: 012,0345
Ⓒ Hexadecimal integer literals:
A sequence of digit (from 0-9 & 10-A, 11-B, 12-C, 13-D,14-E, 15-F) preceded by ox or OX is taken to be a hexadecimal number.Eg: 0xAB,0X1,0XCD3 etc.
Q12: What are the different ways to represent floating-point literals?
Answer: Floating-point literals are numbers having a fractional part. These may be written in one of the two forms called fractional form and exponent form.
(a) Fractional form: It consists of signed or unsigned digits including a decimal point between digits for example 2.0, -13.0, 0.7
(b) Exponent form: It consists of two parts: mantissa and exponent. Eg: 5.8 will be written as 0.58*10 = 0.58E01, where 0.58 is mantissa and 1 is exponent.
Q13: What are delimiters?
Answer: A delimeter is a sequence of one or more characters used to specify the boundary between separate independent regions in plain text or other data streams. The most common delimiters are () , {} , [] , @ , # , : , = etc.
Q14: What are the operators?
Answer: They are the tokens that trigger some computations when applied to variables. There can be unary or binary. Unary operator requires one operand whereas binary operators require two operands. For example, +a (unary operator +), a + b (binary operator +)
Q15: List different types of operators.
Answer: Different types of operator are:
Relational operator (< , > , >= , <= , == , !=)
Logical operator (and , or)
Assignment operator(= , /= , += , *= , -=)
Membership operator( in , not in )
Q16: What are operands?
Answer: The operations are represented by operators and the objects of the operation are referred to as operands.
e.g. a + b is an operation in which a and b are operands added together by + (binary) operator.
Q17: What is a variable?
Answer: Variable represents labelled storage locations whose value can be manipulated during the program run.
For example, x= 5
Here x is a variable, = is the assignment operator. variable x is assigned value as numeric 5.
Q18: What is dynamic typing?
Answer: Consider the following Python snippet:
x=10
print(x)
x = “HELLO”
print(x)
The output is:
10
HELLO
Here variable x is holding integer and later it holds string variable. Dynamic typing is only supported by python.
Q19: What is an assignment in Python?
Answer: In python, we can assign the same value to multiple variables in a single statement and multiple values to multiple variables in a single statement.
eg,
a=b=c=10
x,y,z =10,20,30
Q20: What are different data types supported in Python?
Answer: A data type identifies a type of data and a set of valid operation for it. They are of different types:
(i) Numbers
(ii) string
(iii) list
(iv) tuple
(v) dictionary
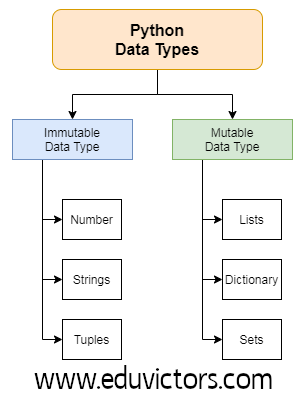
Integers are again of two types: integers (signed) and Boolean (true or false).
The string data type can hold any type of known characters i.e. letters, characters and special characters .ex: ”123”.”abss” etc.
List data type a group of comma-separated value of any data type between square brackets
eg,
[1,2,3] , [‘a’,’b’,’c’] etc.
Tuples: It is a group of comma-separated values of any data type within parentheses.
eg,
p= (1, 2, 3), q= (‘a’, ‘e’, ‘l’)
Dictionaries: it is an unordered set of comma-separated key: value pairs, within {}.
eg,:
{‘a’:1, ‘e’:2, ‘I’:3}
Q21: What is the difference between Mutable and Immutable data types?
Answer: Data objects or Data types can be mutable and immutable types. Mutable data types that can change their value whereas immutable data type can never change their value in place.
e.g.: list , dictionary ,set(mutable)
Q22: What is an expression? List the different types of expressions supported in python are:
Answer:
An expression in python is any valid combination of operators and atoms.
Different types of expressions in Python are:
(1) Arithmetic expression: It Involves numbers and arithmetic operators. For example 2+3**5
(2) Relational expression: It involves variables and relation operators. For example: X >Y , y!=z
(3) Logical expression: It involves variables and logical operators. For example a or b
(4) String expression: only two operators * and + can be applied on strings.
For example: “and” + “then” will print and then
“and” + 2 will print andand.
In a mixed arithmetic expression python converts all operands up to the type of the largest operand which is called type promotion.
Q23: List the different types of statement flow controls are supported in Python.
Answer:
(1) Simple statement: any single executable statement is called a simple statement. For example: a = 10
(2) Empty statement: A statement which does nothing is an empty statement. It takes the following form :
Pass
(3) Compound statement: It is a group of statement executed as a unit.
The Conditionals and the loops are compound statements.
The conditional statement includes if statement,if-else statement and if-elif statement.
Loop is of two types: while loop and for loop.
Q24: What is the syntax of if-statement. Give an example.
Answer:
syntax :
if <condition>:
statement
Example:
if grade==’A’:
print(“well done”)
Q25: What is the syntax of the if-else statement. Give an example.
Answer:
syntax:
if<condition> :
statement
else:
statement
Example:
if grade == ‘A’ :
print (“well done”)
else:
print (”try again”)
Q26: What is the syntax of If-elif statement. Give an example.
Answer:
if <condition>:
statement
elif <condition>:
statement
else:
statement
Example :
num=int(input(“enter the number”))
If num<0:
print(“Invalid entry. Valid range is 0 to 999.’’)
elif num<10:
print(“single digit number is entered”)
elif num<100:
print(“double digit number is entered”)
elif num<=999:
print(“three digit number is entered”)
else:
print(“invalid entry.valid range is 0 to 999.”)
Sample output of this program;
Enter a number (0..999) : -3
Invalid entry. Valid range is 0 to 999.
Q27: Give the syntax of looping statement using for-loop and while-loop. Give examples.
Answer:
for-loop syntax:
for variable in range
statement
Example:
for value in range (3,18):
print(val)
Will print the value from the 3 to 17
while loop syntax:
while <logical expression>
Statement
Example:
count=1
while(count<=10)
print(count)
count=count +1
The output will print values from 1 to 10
Q28: What is the difference between break and continue?
Answer: break and continue are jump statements.
A break statement skips the rest of the loop and jumps over to the statement following the loop. The continue statement is used to skip code within a loop for certain iterations of the loop.
Example:
print( “the loop with break will give output as”)
for I in range (1,11):
if i%3 == 0:
break
else:
print(i)
Output: The loop with break will give output as
1
2
print(“the loop with continue will give output as “)
for I in range(1,11) :
if I %3 ==0:
continue
else:
print(i)
Output: The loop with continue will give output as
1
2
4
5
7
8
10
Q29: WAP to calculate the factorial of a number.
Answer:
num=int(input(“Enter a number”)
fact =1
a= 1
while a<= num :
fact *= a
a += 1
print("The Factorial is ",fact)
Q30: What is a nested loop? Give an example.
Answer: A loop may contain another loop in its body. This form of a loop is called nested loop.
Example:
for i in range(1,6):
for j in range(1,i):
print(“*”,end= ‘ ‘)
print()
Output:
*
* *
* * *
* * * *
☞See also:
Important Abbreviations To Memorise
Networking (Q & A)
Networking - Data Transmission Media (Q & A)
Informatics Practices (2016-17) - Delhi Region - Question Paper
Database Concepts (Important Terms)
IT Applications (Q & A)
SQL Commands (Summary)
HTML Basics (1 Mark Based Q & A)
What is XML? (Q & A)
Database Concepts (Important Terms)
Divisibility Rules
SQL Commands (Summary)
SQL Commands (Summary)
HTML Basics (1 Mark Based Q & A)
What is XML? (Q & A)
Database Concepts (Important Terms)
Divisibility Rules
SQL Commands (Summary)
No comments:
Post a Comment
We love to hear your thoughts about this post!
Note: only a member of this blog may post a comment.